Allstate NI Coding Competition — Challenge 1
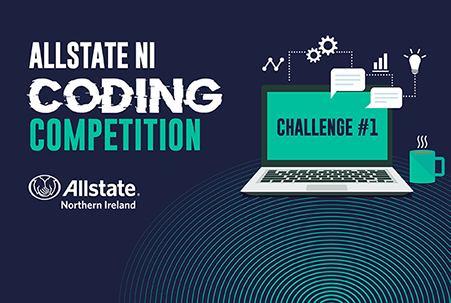
Enter our new competition for the chance to win £50!
Can you spot the error in the code below? If you can, you could win £50! Simply find and fix the error, then click the button below to fill out the form to be in with a chance of winning. Identify the error and, if you're correct, your name will be added to our prize draw. Three lucky contestants will be drawn and win the prize! Do you have what it takes to be one of them?
import java.util.Arrays;
/**
* The Connect4 class is a basic simulator for the popular
* Connect4 game where opposing players alternately
* drop a coloured counter into a 6 x 7 grid seeking
* to achieve 4 of their counters resting in a horizontal,
* vertical or diagonal row before their opponent.
*
* In game play, rows and columns are always
* referenced 1-6 or 1-7 appropriately
* @version 1.0, Apr 2021
*/
1 public class Connect4 {
2 private final char[][] gameBoard = {
3 {'0', '0', '0', '0', '0', '0', '0'}, //Row 1, Bottom
4 {'0', '0', '0', '0', '0', '0', '0'}, //Row 2
5 {'0', '0', '0', '0', '0', '0', '0'}, //Row 3
6 {'0', '0', '0', '0', '0', '0', '0'}, //Row 4
7 {'0', '0', '0', '0', '0', '0', '0'}, //Row 5
8 {'0', '0', '0', '0', '0', '0', '0'} //Row 6, Top
/** @param counter - A single character denoting the colour of the counter played
* @param col - The number of the column into which the counter is played - relative from 1 */
9 public void playCounter(final char counter, final int col) {
10 for(int row = 1; row <= gameBoard.length; row++) {
11 if(isSlotEmpty(col, row)) {
12 gameBoard[row-1][col-1] = counter;
13 return;
14 }
15 }
16 }
/**
*
* @return - A boolean value indicating if the last move has won the game
*/
17 public boolean isGameWon() {
18 if(fourInARowHorizontally()) return true;
19 if(fourInARowVertically()) return true;
20 if(fourInARowDiagonallyLeftToRight()) return true;
21 if(fourInARowDiagonallyRightToLeft()) return true;
22 return false;
23 }
24 public char getCounter(final int col, final int row) {
25 return gameBoard[row-1][col-1];
26 }
27 private boolean isSlotEmpty(final int col, final int row) {
28 return getCounter(col, row) == '0';
29 }
30 private boolean fourInARowHorizontally() {
31 for(int row = 1; row <= gameBoard.length; row++) {
32 int numInARow = 1;
33 for(int col = 1; col <= gameBoard[row-1].length-1; col++) {
34 if(isSlotEmpty(col, row)) {
35 numInARow = 1;
36 } else if(getCounter(col, row) == getCounter(col+1, row)) {
37 numInARow++;
38 if(numInARow == 4) return true;
39 } else
40 numInARow = 1;
41 }
42 }
43 return false;
44 }
45 private boolean fourInARowVertically() {
46 for(int col = 1; col <= gameBoard[0].length; col++) {
47 int numInARow = 1;
48 for(int row = 1; row <= gameBoard.length; row++) {
49 if(isSlotEmpty(col, row)) {
50 numInARow = 1;
51 } else if(getCounter(col, row) == getCounter(col, row+1)) {
52 numInARow++;
53 if(numInARow == 4) return true;
54 } else
55 numInARow = 1;
56 }
57 }
58 return false;
59 }
60 private boolean fourInARowDiagonallyLeftToRight() {
61 for(int row = 1; row <= 3; row++) {
62 for(int col = 1; col <= 4; col++) {
63 if(!isSlotEmpty(col, row)) {
64 if((getCounter(col, row) == getCounter(col+1, row+1))
65 && (getCounter(col+1, row+1) == getCounter(col+2, row+2))
66 && (getCounter(col+2, row+2) == getCounter(col+3, row+3))
67 ) return true;
68 }
69 }
70 }
71 return false;
72 }
73 private boolean fourInARowDiagonallyRightToLeft() {
74 for(int row = 1; row <= 3; row++) {
75 for(int col = 4; col <= 7; col++) {
76 if(!isSlotEmpty(col, row)) {
77 if((getCounter(col, row) == getCounter(col-1, row+1))
78 && (getCounter(col-1, row+1) == getCounter(col-2, row+2))
79 && (getCounter(col-2, row+2) == getCounter(col-3, row+3))
80 ) return true;
81 }
82 }
83 }
84 return false;
85 }
86 }
Enter to win here